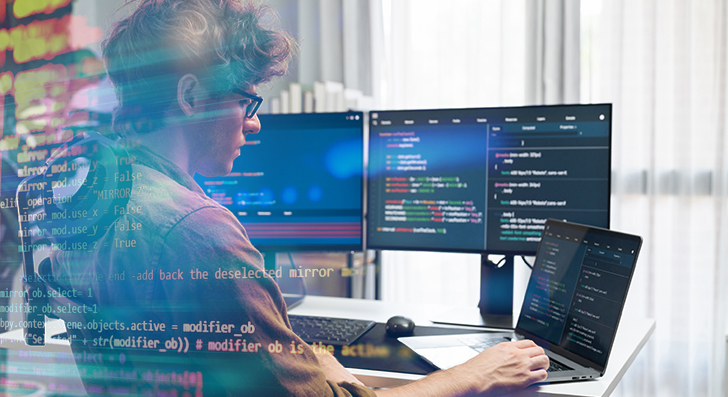
Scalability usually means your application can deal with growth—extra people, far more info, and a lot more site visitors—with out breaking. As a developer, making with scalability in mind saves time and anxiety later. Below’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it ought to be aspect of the plan from the start. Many apps fail if they expand quickly since the first layout can’t handle the extra load. To be a developer, you must think early about how your system will behave stressed.
Commence by designing your architecture to get adaptable. Stay away from monolithic codebases where by every little thing is tightly related. As an alternative, use modular style or microservices. These designs crack your app into more compact, unbiased parts. Every single module or company can scale on its own without the need of impacting The complete system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 customers or maybe 100? Choose the proper variety—relational or NoSQL—based on how your info will increase. Approach for sharding, indexing, and backups early, even if you don’t have to have them yet.
Yet another significant issue is to stay away from hardcoding assumptions. Don’t compose code that only performs underneath present-day circumstances. Give thought to what would happen Should your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use layout designs that guidance scaling, like message queues or occasion-driven techniques. These support your app take care of far more requests devoid of finding overloaded.
After you Develop with scalability in mind, you're not just preparing for success—you're decreasing long term headaches. A well-prepared procedure is less complicated to take care of, adapt, and improve. It’s greater to organize early than to rebuild later on.
Use the proper Database
Deciding on the appropriate database is often a crucial A part of building scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or perhaps trigger failures as your application grows.
Start out by comprehension your information. Can it be hugely structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. They also assist scaling methods like browse replicas, indexing, and partitioning to deal with extra traffic and knowledge.
In case your facts is more adaptable—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and may scale horizontally additional effortlessly.
Also, look at your read and publish styles. Have you been doing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy compose load? Check into databases that can manage significant write throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need State-of-the-art scaling options now, but choosing a database that supports them implies you gained’t have to have to modify later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your information according to your accessibility patterns. And often keep an eye on database functionality while you increase.
Briefly, the appropriate databases depends upon your app’s structure, velocity requires, And exactly how you hope it to mature. Choose time to select correctly—it’ll help save a great deal of hassle afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can decelerate general performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Commence by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t pick the most advanced Resolution if a simple a person will work. Keep your capabilities limited, targeted, and straightforward to check. Use profiling tools to find bottlenecks—places wherever your code normally takes as well extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish factors down more than the code by itself. Make sure Just about every query only asks for the information you truly want. Avoid Pick *, which fetches everything, and alternatively select certain fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
In the event you observe the same info staying requested repeatedly, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred documents might crash after they have to deal with 1 million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to manage additional people plus more targeted visitors. If everything goes through 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these applications enable keep the application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Instead of one server accomplishing the many get the job done, the load balancer routes end users to distinct servers depending on availability. This means no single server gets overloaded. If just one server goes down, the load check here balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers request a similar facts once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. It is possible to serve it through the cache.
There are two prevalent varieties of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your app additional efficient.
Use caching for things which don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are basic but impressive resources. Jointly, they help your app tackle much more end users, keep fast, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application develop very easily. That’s wherever cloud platforms and containers are available. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to acquire hardware or guess foreseeable future ability. When website traffic improves, you could add much more sources with just a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide providers like managed databases, storage, load balancing, and safety instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your app in between environments, from a notebook for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, and that is great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies you can scale rapidly, deploy easily, and Get well quickly when complications take place. If you want your app to mature with out boundaries, start working with these resources early. They help save time, reduce threat, and assist you stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Portion of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for vital problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, normally right before people even observe.
Monitoring is also practical after you make alterations. Should you deploy a fresh feature and find out a spike in faults or slowdowns, you may roll it back again before it results in true harm.
As your application grows, targeted traffic and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a strong foundation. By designing very carefully, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase effortlessly without having breaking stressed. Start tiny, think massive, and Establish intelligent.