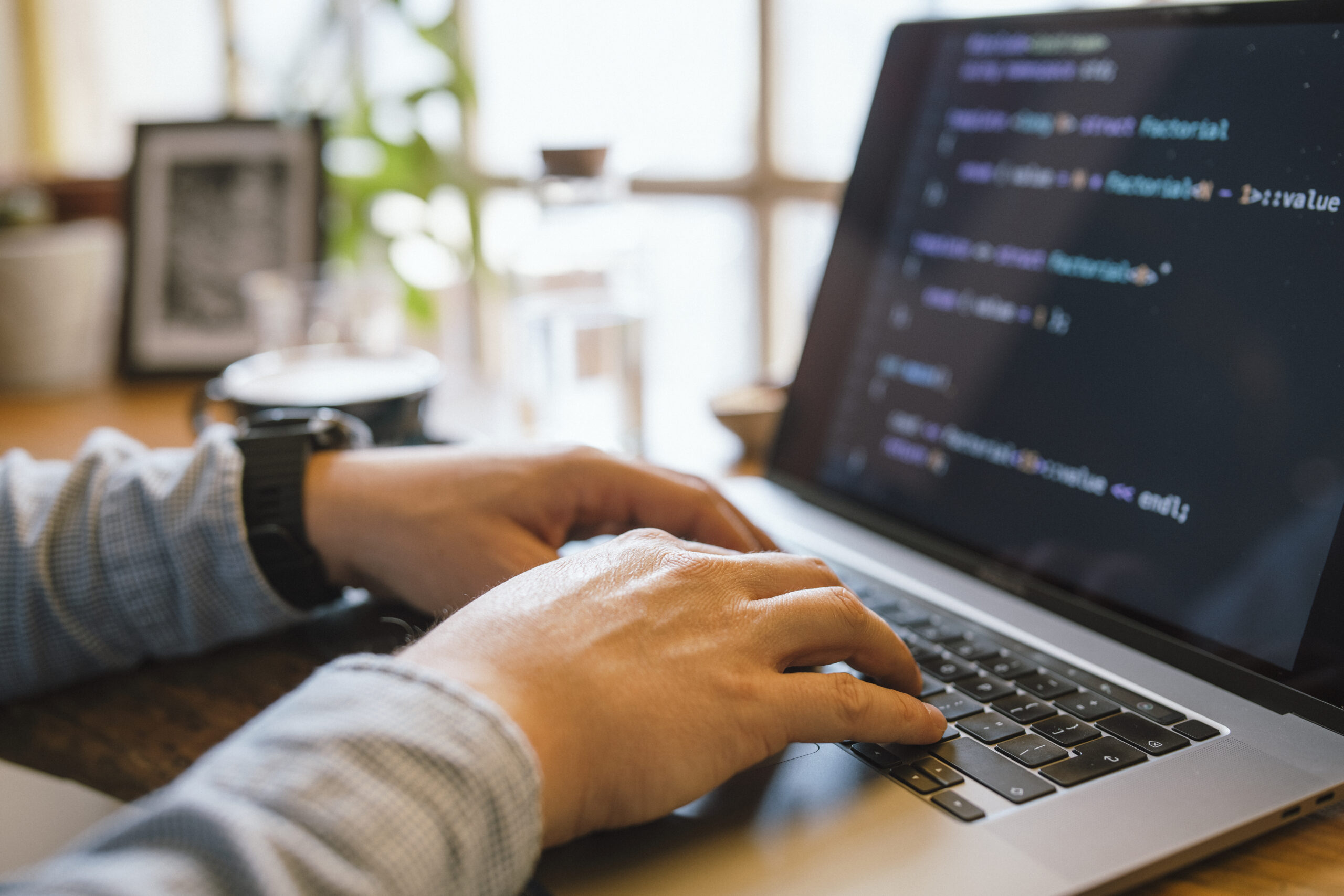
Debugging is Probably the most necessary — yet frequently neglected — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Feel methodically to solve difficulties successfully. Whether you are a starter or a seasoned developer, sharpening your debugging abilities can save hours of aggravation and substantially enhance your efficiency. Here are several procedures that will help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest ways builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although creating code is 1 part of enhancement, figuring out the way to interact with it correctly through execution is equally essential. Fashionable progress environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these instruments can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and perhaps modify code about the fly. When used effectively, they Allow you to notice precisely how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, observe network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into manageable tasks.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning processes and memory management. Finding out these applications could have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate systems like Git to know code historical past, come across the precise instant bugs were introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about building an intimate understanding of your advancement setting making sure that when challenges crop up, you’re not shed in the dark. The better you know your tools, the greater time you could expend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most essential — and sometimes ignored — actions in efficient debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation the place the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of opportunity, often bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise problems under which the bug happens.
Once you’ve gathered sufficient facts, attempt to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the edge circumstances or point out transitions involved. These exams don't just assist expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be surroundings-precise — it'd occur only on specified functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms might be instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a mindset. It needs persistence, observation, and also a methodical solution. But once you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes securely, and talk a lot more Obviously using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s where builders prosper.
Examine and Have an understanding of the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Erroneous. In lieu of looking at them as discouraging interruptions, builders must find out to treat error messages as immediate communications with the process. They generally inform you just what occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in total. Many builders, especially when less than time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root induce. Don’t just copy and paste mistake messages into serps — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized issues and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Just about the most strong equipment in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with knowing what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts during enhancement, Details for standard functions (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant facts. An excessive amount logging can obscure crucial messages and slow down your process. Give attention to important situations, condition modifications, enter/output values, and significant choice details within your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular useful in output environments the place stepping through code isn’t doable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about stability and clarity. Which has a nicely-considered-out logging approach, it is possible to lessen the time it will take to spot challenges, acquire deeper visibility into your purposes, and improve the In general maintainability and dependability of your code.
Think Just like a Detective
Debugging is not just a specialized task—it's a type of investigation. To correctly recognize and take care of bugs, developers need to tactic the process like a detective fixing a thriller. This mindset assists stop working advanced challenges into workable elements and observe clues logically to uncover the foundation cause.
Commence by gathering evidence. Look at the indicators of the situation: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, acquire as much pertinent data as you may devoid of leaping to conclusions. Use logs, take a look at conditions, and person studies to piece collectively a transparent image of what’s taking place.
Subsequent, type hypotheses. Request oneself: What may be triggering this habits? Have any alterations just lately been manufactured on the codebase? Has this concern occurred before less than identical instances? The intention will be to slim down prospects and discover likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for future troubles and assistance Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Create Assessments
Producing checks is among the most effective methods to increase your debugging techniques and In general development efficiency. Tests not merely support capture bugs early and also function a security Internet that provides you self esteem when earning changes for your codebase. A effectively-examined application is simpler to debug as it lets you pinpoint particularly wherever and when a problem occurs.
Start with unit checks, which deal with individual capabilities or modules. These compact, isolated tests can quickly reveal whether or not a specific piece of logic is working as expected. Any time a exam fails, you promptly know where by to glimpse, noticeably lessening some time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, combine integration exams and finish-to-end checks into your workflow. These support make certain that different elements of your application work alongside one another efficiently. They’re especially helpful for catching bugs that occur in advanced programs with numerous factors or providers interacting. If something breaks, your assessments can tell you which Portion of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to definitely Believe critically regarding your code. To test a element correctly, you would like to grasp its inputs, envisioned outputs, and edge circumstances. This volume of knowing naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. When the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture much more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough issue, it’s straightforward to be immersed in the situation—gazing your monitor for hours, attempting Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see The difficulty from a new perspective.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your Mind results in being fewer economical at challenge-fixing. A short wander, a espresso split, or perhaps switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report getting the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support stop burnout, especially all through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent enables you to return with renewed Electrical power plus a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for 45–sixty minutes, then have a 5–ten moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging Eventually.
In short, using breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug click here you encounter is more than just A brief setback—It is really an opportunity to develop like a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a thing important in the event you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a few important queries after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code evaluations, or logging? The solutions usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After a while, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick awareness-sharing session, serving to Other individuals steer clear of the similar concern boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are usually not the ones who generate ideal code, but individuals that constantly study from their errors.
In the long run, each bug you correct provides a brand new layer on your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you happen to be knee-deep inside of a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.